Cards
We think cards are one of the best ways to organise your information. We went all out with possibilities and we recommended finding the right fit for your product.
import {StatsCard} from '@/components'
import {ChartCard} from '@/components'
Local Usage
components: {
ChartCard,
StatsCard
}
Global Usage
Vue.component(ChartCard)
Vue.component(StatsCard)
Stats card
update
Just Updated
StatsCard Slots
Name | Description |
---|---|
header | Default card header |
footer | Content for card footer |
Chart card
For the implementation of graphic charts, we used Chartist.js.
Website Views
Last Campaign Performance
access_time
updated 10 days ago
ChartCard Attributes
Attribute | Description | Type | Accepted values | Default |
---|---|---|---|---|
type | Chart type | string | line/bar/pie | - |
options | Chart options | string | - | - |
data | Chart data | string | - | - |
title | Card title | string | - | — |
subTitle | Card subtitle (displayed above with smaller font) | string | - | - |
ChartCard Slots
Name | Description |
---|---|
default | Default card content |
footer | Content for card footer |
Lock card
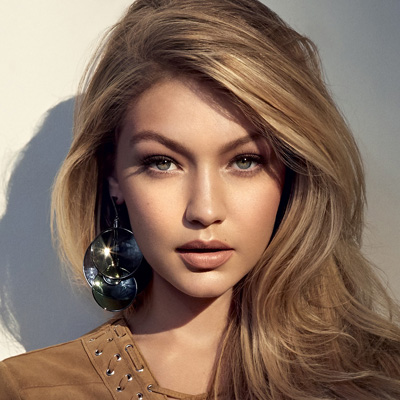
Tania Andrew
LockCard Slots
Name | Description |
---|---|
imageProfile | Default card image |
title | Default card title |
content | Default card content |
footer | Content for card footer |
Login card
Log in
Or Be Classical
face
email
lock_outline
LoginCard Slots
Name | Description |
---|---|
title | Default card title |
description | Default card description |
buttons | card buttons |
footer | Content for card footer |
Pricing card
Freelancer
Free
This is good if your company size is between 2 and 10 Persons.
PricingCard Slots
Name | Description |
---|---|
category | card category |
icon | Default card icon |
title | Default card title |
description | Default card description |
footer | Content for card footer |
Product card
We created a beautiful animation for this type of card. By default this animation is set on true
, if you want to disable it you can set the Attribute header-animation
to false
.
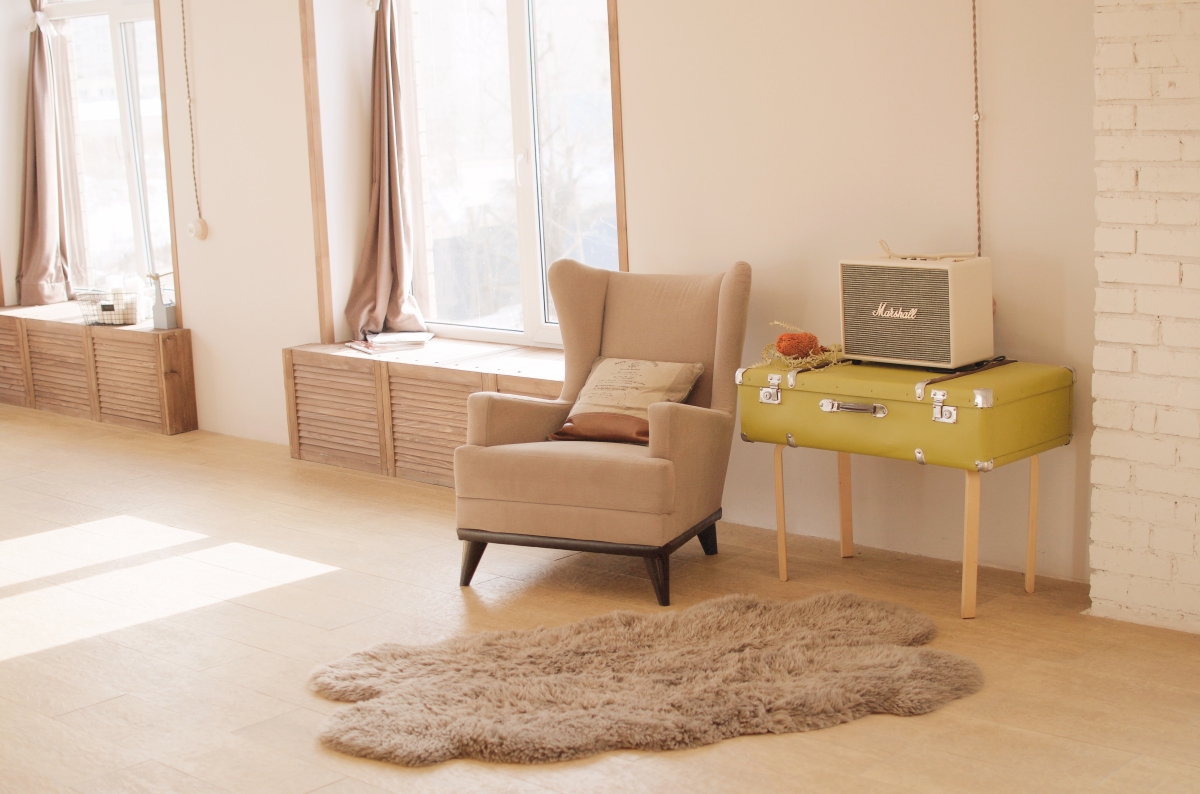
Cozy 5 Stars Apartment
The place is close to Barceloneta Beach and bus stop just 2 min by walk and near to "Naviglio" where you can enjoy the main night life in Barcelona.
$899/night
place Barcelona, Spain
ProductCard Slots
Name | Description |
---|---|
imageHeader | Default card image |
fixed-button | card fixed button |
first-button | card details buttons |
second-button | card details buttons |
third-button | card details buttons |
title | Default card title |
description | Default card description |
footer | Content for card footer |
Testimonial card
TestimonialCard Slots
Name | Description |
---|---|
header-icon | card header icon |
content | card content |
footer | card footer |