# Cards
Bootstrap's cards provide a flexible and extensible content container with multiple variants and options.
import {Card} from 'src/components'
# Local usage
components: {
Card
}
2
3
# Global usage
Vue.component(Card)
# Example
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
# Content types
# Titles, text, and links
Card titles are used by adding .card-title
to a <h*>
tag.
In the same way, links are added and placed next to each other by adding .card-link
to an <a>
tag.
Subtitles are used by adding a .card-subtitle
to a <h*>
tag.
If the .card-title
and the .card-subtitle
items are placed in a .card-body
(default slot contains a card-body
class) item, the card title and subtitle are aligned nicely.
Card title
Card subtitle
Some quick example text to build on the card title and make up the bulk of the card's content.
Card link Another link# Images
.card-img-top
places an image to the top of the card. With .card-text
, text can be added to the card.
Text within .card-text
can also be styled with the standard HTML tags.
Some quick example text to build on the card title and make up the bulk of the card's content.
# List groups
Create lists of content in a card with a flush list group.
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
# Header and footer
Special title treatment
With supporting text below as a natural lead-in to additional content.
# Quote card
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat a ante.
# Text alignment
Special title treatment
With supporting text below as a natural lead-in to additional content.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Special title treatment
With supporting text below as a natural lead-in to additional content.
# Plain card
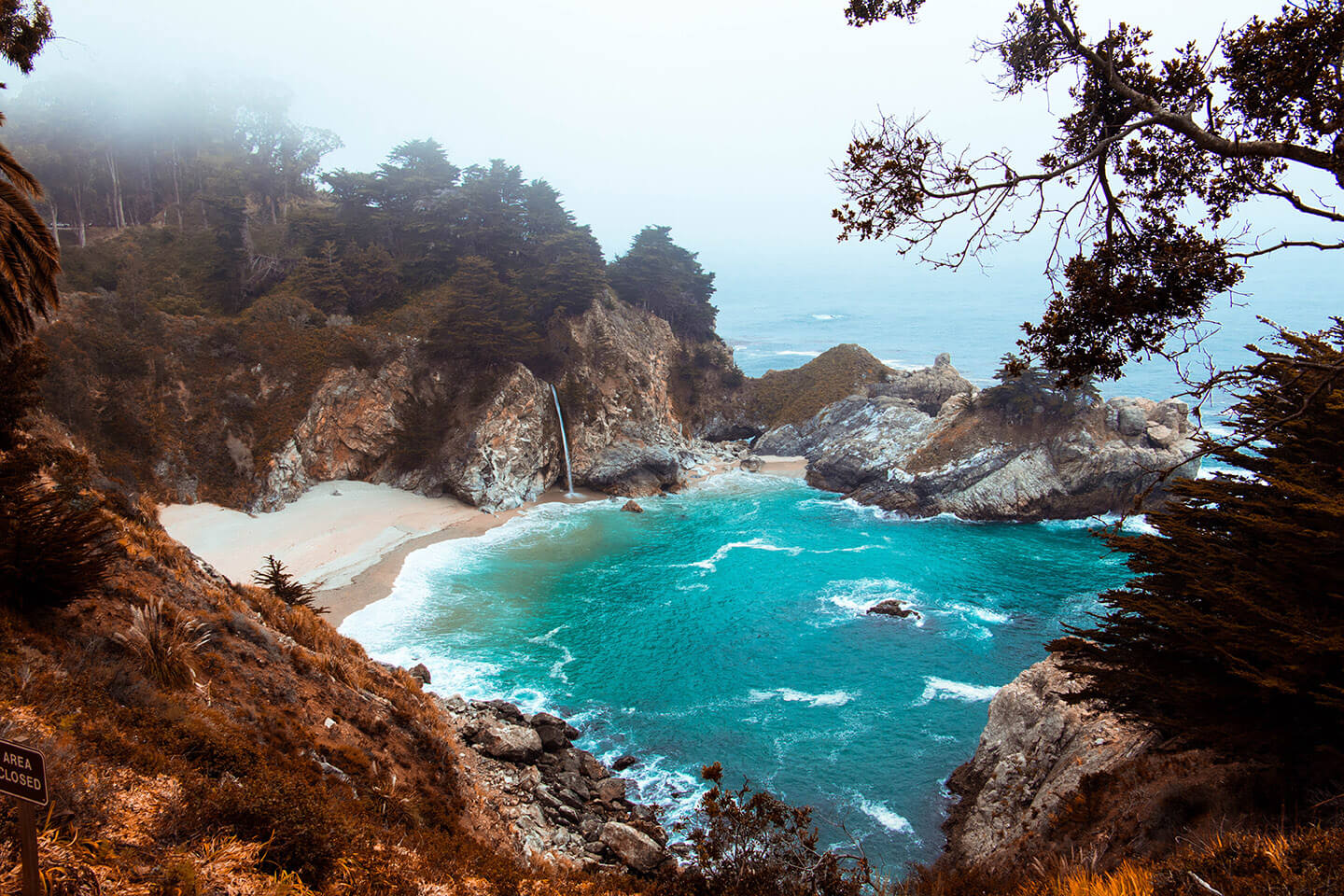
Focus
Stay Focused: Train Your Brain
Our brains are finely attuned to distraction, so today's digital environment makes it especially hard to focus...
# Card Slots
Name | Description |
---|---|
default | Content for card body |
header | Content for card header |
image | Content for card image (displayed above header) |
avatar | Content for card avatar (displayed above header) |
info | Content for card info (displayed above header) |
raw-content | Slot for content (displayed below body) |
footer | Content for card footer |
← Button Checkboxes →