# Cards
Bootstrap's cards provide a flexible and extensible content container with multiple variants and options.
# Examples
import {Card} from 'src/components'
Global usage
Vue.component(Card.name, Card)
For local usage
export default {
components: {
Card
}
}
Below is an example of a basic card with mixed content and a fixed width. Cards have no fixed width to start, so they’ll naturally fill the full width of its parent element. This is easily customized with our various sizing options.
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhere# Content types
Cards support a wide variety of content, including images, text, list groups, links, and more. Below are examples of what’s supported.
# Body
The building block of a card is the default slot
. Just put content in the <card>
component
and it will be wrapped in a div with .card-body
class.
Use it whenever you need a padded section within a card.
# Titles, text, and links
Card titles are used by passing title
prop or by using the slot="header"
or
by using the .card-title
class.
Links are added and placed next to each other by adding .card-link to an <a>
tag.
Subtitles are used by adding a .card-subtitle
to a <h*>
tag.
If the .card-title
and the .card-subtitle
items are placed in a
default slot (card body)
item, the card title and subtitle are aligned nicely.
Card title
Card subtitle
Some quick example text to build on the card title and make up the bulk of the card's content.
Card link Another link# Images
.card-img-top
along with slot="image"
places an image to the top of the card.
With .card-text
, text can be added to the card.
Text within .card-text
can also be styled with the standard HTML tags.
Some quick example text to build on the card title and make up the bulk of the card's content.
# List groups
Create lists of content in a card with a flush list group.
You have to use the slot="raw-content"
to avoid padding for the list group items
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
# Header and footer
Add an optional header and/or footer within a card with the help of footer
and header
slots.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere# Quote card
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat a ante.
# Center aligned
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere# Text alignment
You can quickly change the text alignment of any card—in its entirety or specific parts—with our text align classes.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhereSpecial title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhereSpecial title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere# Image caps
Similar to headers and footers, cards can include top and bottom “image caps”—images at the top or bottom of a card.
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
# Image overlays
Turn an image into a card background and overlay your card’s text. Depending on the image, you may or may not need additional styles or utilities.
# Colors
Set for.card-white
class to change the card background’s color.
This class is available only for the following cards: card-pricing, card-login, card-signup
.
pro
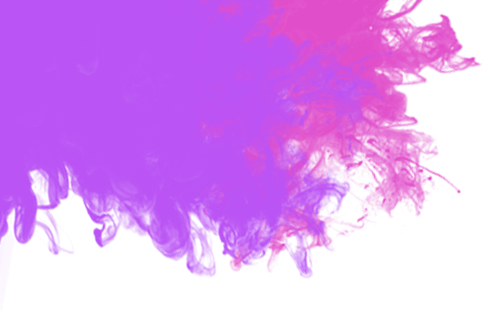
- 300 messages
- 150 emails
- 24/7 Support
$95
95
Professional plan
basic
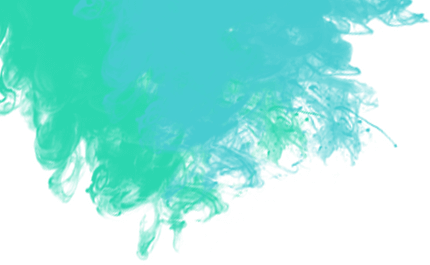
- 50 messages
- 100 emails
- 24/7 Support
$57
57
Basic plan
# Cards props
# Cards Slots
Slot | Description |
---|---|
default | Default content for card body |
header | Card header content |
footer | Card footer content |
raw-content | Slot in case you need full control over card content (no header, footer, body) |
image | Card image placed on header |
image-bottom | Card image placed on bottom of the card |