Cards
We've created three types of cards, one is for simple use, one is for creating stats cards and the last one is for creating chart cards. They have to be imported where you use them like this:
import {Card, StatsCard, ChartCard} from '@/components'
Local usage
components: {
Card,
StatsCard,
ChartCard
}
Global usage
Vue.component(Card)
Vue.component(StatsCard)
Vue.component(ChartCard)
Cards
TIP
Cards are a very simple and intuitive way of displaying rich content
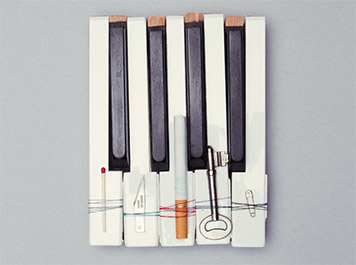
Stats cards
TIP
Stats cards are a nice way to display visually important statistics in your dashboard such as number of online users, log count, error count or other relevant information.
Capacity
105GB Updated Now
Chart cards
TIP
Chart cards are small wrapper cards over Chartist library
2015 Sales
All products including Taxes
Card Attributes
Attribute | Description | Type | Accepted values | Default |
---|---|---|---|---|
title | Card title | string | - | — |
subTitle | Card subtitle (displayed above with smaller font) | string | - | - |
Card Slots
Name | Description |
---|---|
header | Content for card header |
content | Default card content |
footer | Content for card footer |
StatsCard Slots
Name | Description |
---|---|
header | Content for card header |
content | Default card content |
footer | Content for card footer |
ChartCard Attributes
Attribute | Description | Type | Accepted values | Default |
---|---|---|---|---|
title | Card title | string | - | — |
subTitle | Card subtitle (displayed above with smaller font) | string | - | - |
footerText | Card footer text | string | - | - |
chartType | Chart type | string | Line | Pie |
chartOptions | Chartist chart options | object | Chartist Options | {} |
chartData | Chartist chart data | object | Chartist Data | {lines: [], series: []} |